网站此前用的回到顶部按钮是通过插件市场中的插件实现,效果还不错,不过功能比较单一。
我在其他的博客中看到了一个可以在页面下拉过程中实现当前页面进度的回顶按钮,觉得实用性很棒,因此尝试通过页面脚本的形式导入。
但是这种方式过于低效,且没法很好自定义,所以便尝试是否可以通过插件的形式导入功能,通过和GPT的一番交流,大致明白了WP插件的制作过程。
WP的插件可以通过Zip文件导入,其中的结构为:
backtop/
├── assets/
│ ├── backtop.css
│ └── backtop.js
├── backtop.php
└── readme.txt (可选)
其中backtop.php是插件的核心文件,包含了插件的主要功能和初始化代码。
backtop.css为样式表,js则是JavaScript 文件,写交互逻辑使用。
参考代码:
PHP:
<?php
/*
Plugin Name: 写你的插件名称
Description: 描述
Version: 版本号
Author: 作者
Author URI: https://wanxuefeiyang.cn
License: GPL2
*/
// Enqueue CSS and JS,注意地址
function backtop_enqueue_assets() {
wp_enqueue_style('backtop-style', plugin_dir_url(__FILE__) . 'assets/backtop.css');
wp_enqueue_script('backtop-script', plugin_dir_url(__FILE__) . 'assets/backtop.js', [], false, true);
$options = get_option('backtop_options');
wp_localize_script('backtop-script', 'backTopOptions', [
'position' => $options['position'] ?? 'bottom-right',
'color' => $options['color'] ?? '#000000',
'size' => $options['size'] ?? '40px',
'shape' => $options['shape'] ?? 'circle',
'margin' => $options['margin'] ?? '20px 20px'
]);
}
add_action('wp_enqueue_scripts', 'backtop_enqueue_assets');
// 插入 HTML
function backtop_render_html() {
echo '
<div id="backtop-tool">
<ul>
<li id="backtop" class="hidden">
<span id="backtop-percentage">0%</span>
</li>
</ul>
</div>';
}
add_action('wp_footer', 'backtop_render_html');
// 设置页面,可以自定义一些内容
function backtop_add_settings_page() {
add_options_page(
'BackTop Settings',
'BackTop',
'manage_options',
'backtop-settings',
'backtop_render_settings_page'
);
}
add_action('admin_menu', 'backtop_add_settings_page');
function backtop_render_settings_page() {
?>
<div class="wrap">
<h1>BackTop Settings</h1>
<form method="post" action="options.php">
<?php
settings_fields('backtop_options_group');
do_settings_sections('backtop-settings');
submit_button();
?>
</form>
</div>
<?php
}
function backtop_register_settings() {
register_setting('backtop_options_group', 'backtop_options', [
'type' => 'array',
'sanitize_callback' => 'backtop_sanitize_options',
'default' => [
'position' => 'bottom-right',
'color' => '#000000',
'size' => '40px',
'shape' => 'circle',
'margin' => '20px 20px'
],
]);
add_settings_section('backtop_main_section', 'Main Settings', null, 'backtop-settings');
add_settings_field('position', 'Position', 'backtop_position_field', 'backtop-settings', 'backtop_main_section');
add_settings_field('color', 'Background Color', 'backtop_color_field', 'backtop-settings', 'backtop_main_section');
add_settings_field('size', 'Button Size', 'backtop_size_field', 'backtop-settings', 'backtop_main_section');
add_settings_field('shape', 'Shape', 'backtop_shape_field', 'backtop-settings', 'backtop_main_section');
add_settings_field('margin', 'Margin', 'backtop_margin_field', 'backtop-settings', 'backtop_main_section');
}
add_action('admin_init', 'backtop_register_settings');
function backtop_position_field() {
$options = get_option('backtop_options');
?>
<select name="backtop_options[position]">
<option value="bottom-right" <?php selected($options['position'], 'bottom-right'); ?>>Bottom Right</option>
<option value="bottom-left" <?php selected($options['position'], 'bottom-left'); ?>>Bottom Left</option>
</select>
<?php
}
function backtop_color_field() {
$options = get_option('backtop_options');
?>
<input type="color" name="backtop_options[color]" value="<?php echo esc_attr($options['color']); ?>">
<?php
}
function backtop_size_field() {
$options = get_option('backtop_options');
?>
<input type="text" name="backtop_options[size]" value="<?php echo esc_attr($options['size']); ?>" placeholder="e.g., 40px">
<?php
}
function backtop_shape_field() {
$options = get_option('backtop_options');
?>
<select name="backtop_options[shape]">
<option value="circle" <?php selected($options['shape'], 'circle'); ?>>Circle</option>
<option value="square" <?php selected($options['shape'], 'square'); ?>>Square</option>
</select>
<?php
}
function backtop_margin_field() {
$options = get_option('backtop_options');
?>
<input type="text" name="backtop_options[margin]" value="<?php echo esc_attr($options['margin']); ?>" placeholder="e.g., 20px 20px">
<?php
}
function backtop_sanitize_options($options) {
$options['position'] = in_array($options['position'], ['bottom-right', 'bottom-left']) ? $options['position'] : 'bottom-right';
$options['color'] = sanitize_hex_color($options['color']);
$options['size'] = preg_match('/^\d+(px|em|%)$/', $options['size']) ? $options['size'] : '40px';
$options['shape'] = in_array($options['shape'], ['circle', 'square']) ? $options['shape'] : 'circle';
$options['margin'] = sanitize_text_field($options['margin']);
return $options;
}
PHP
JS:
(function () {
const backTopTool = document.getElementById("backtop-tool");
const backTopButton = document.getElementById("backtop");
const percentageDisplay = document.getElementById("backtop-percentage");
if (backTopTool && backTopButton) {
const { position, color, size, shape, margin } = backTopOptions || {};
const [vertical, horizontal] = position.split("-");
const [marginY, marginX] = margin.split(" ");
backTopTool.style[vertical] = marginY || "20px";
backTopTool.style[horizontal] = marginX || "20px";
backTopButton.style.backgroundColor = color || "#000";
backTopButton.style.width = size || "40px";
backTopButton.style.height = size || "40px";
backTopButton.style.borderRadius = shape === "circle" ? "50%" : "0";
percentageDisplay.style.fontSize = `${Math.max(parseInt(size) * 0.4, 8)}px`;
}
const updateScrollProgress = () => {
const scrollTop = window.scrollY;
const scrollHeight = document.documentElement.scrollHeight - document.documentElement.clientHeight;
const scrollPercentage = Math.round((scrollTop / scrollHeight) * 100);
if (percentageDisplay) {
percentageDisplay.innerHTML = scrollPercentage >= 95 ? "▲" : `${scrollPercentage}%`;
}
if (backTopButton) {
backTopButton.classList.toggle("hidden", scrollTop < 200);
}
};
const scrollToTop = () => {
window.scrollTo({ top: 0, behavior: "smooth" });
};
backTopButton?.addEventListener("click", scrollToTop);
window.addEventListener("scroll", updateScrollProgress);
})();
JavaScript
CSS:
#backtop-tool {
position: fixed;
z-index: 9999;
}
#backtop-tool ul {
list-style: none;
padding: 0;
margin: 0;
}
#backtop-tool .hidden {
display: none;
}
#backtop-tool li {
display: flex;
justify-content: center; /* 水平居中 */
align-items: center; /* 垂直居中 */
width: var(--size, 40px);
height: var(--size, 40px);
background: var(--color, rgba(0, 0, 0, 0.7));
color: #fff;
border-radius: var(--shape, 50%);
cursor: pointer;
font-size: calc(var(--size, 40px) * 0.4); /* 根据按钮大小动态调整字体 */
text-align: center; /* 对齐数字文本 */
overflow: hidden;
box-sizing: border-box;
}
CSS
管理界面如下:
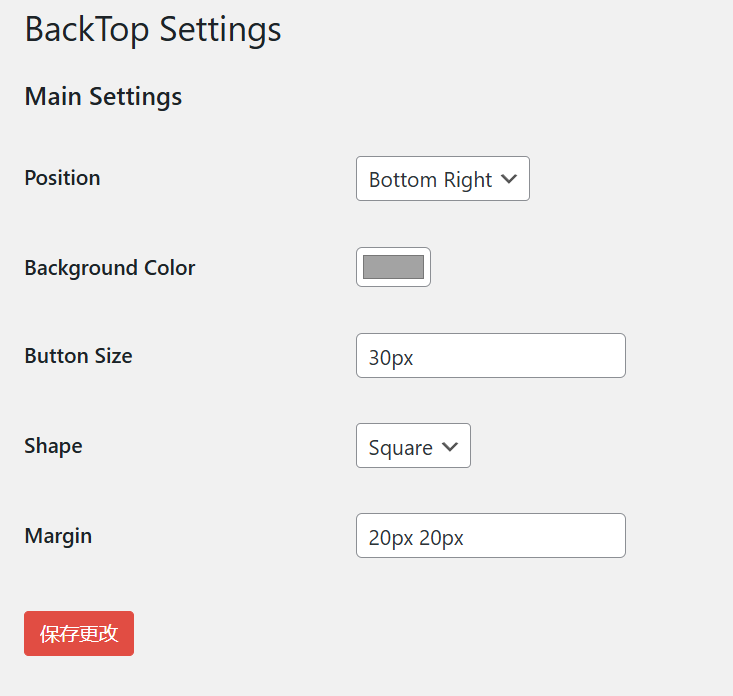
从插件制作到实现的过程,给我的感觉是通过插件对页面、功能进行修改,可以避免我们干扰WP核心文件,让页面中的其他功能不受影响,对灵活性、安全性都有一定的增强,这对于我这种半桶水来说非常利好,即便哪里设置错了直接把插件删了就好嘛,可玩性很高。
发表回复